|
| 1 | +# CPP-Notes |
| 2 | +C++ Notes |
| 3 | + |
| 4 | +## 1 Requirements |
| 5 | + |
| 6 | +This tutorial is based on C++11. Make sure you are using the latest IDE's - XCode (Mac) or Visual Studio (Windows). You can use other IDE's but make sure it supports the latest C++ version. |
| 7 | + |
| 8 | +### 1.1 Mac |
| 9 | + |
| 10 | +**Adding files to Xcode** |
| 11 | + |
| 12 | +1. Download Xcode from the App Store. |
| 13 | +2. Open Xcode application and click on `File > New > Workspace...`. |
| 14 | +3. Name the `Workspace` as `CPP-Notes`. |
| 15 | +4. Click on the `+` sign and then click on `Add Files to "CPP-Notes"...` |
| 16 | + 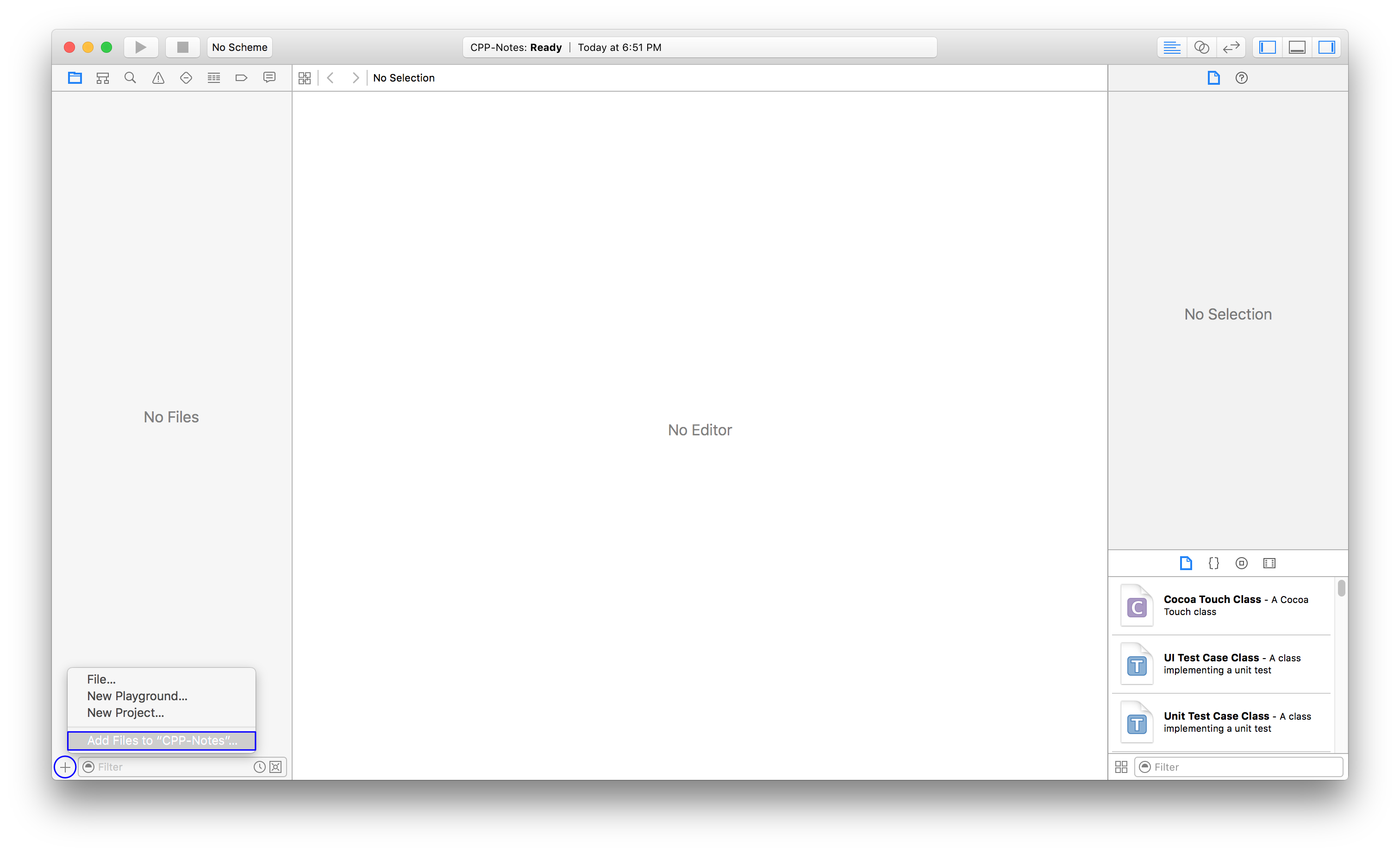 |
| 17 | + |
| 18 | +**Creating Project** |
| 19 | + |
| 20 | +1. Click on the `+` sign and then click on `New Project...` |
| 21 | + 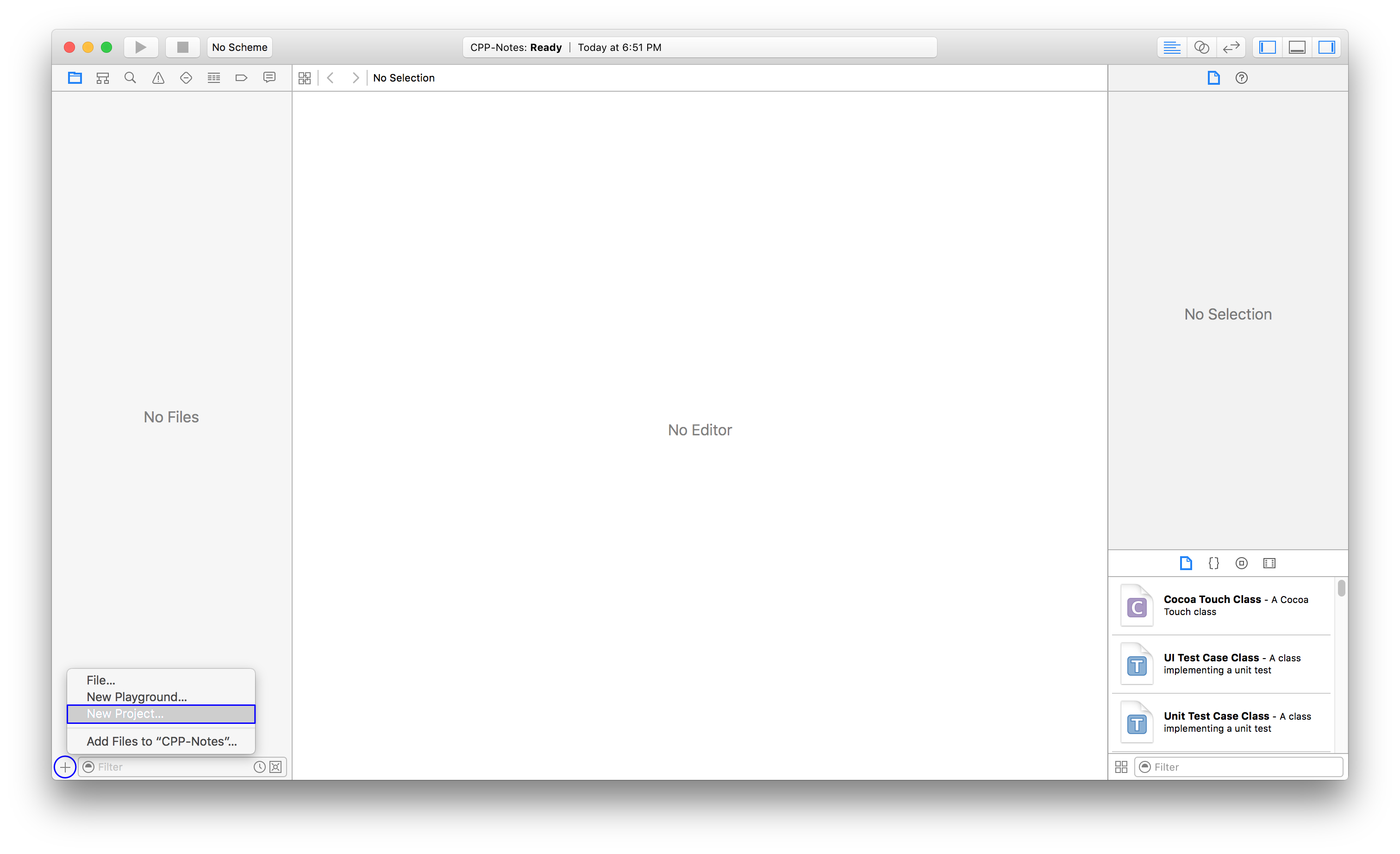 |
| 22 | +2. Under `OSX > Application` select `Command Line Tool` and click next. In that, type in the name of the product and make sure you select the language as `C++`. |
| 23 | + 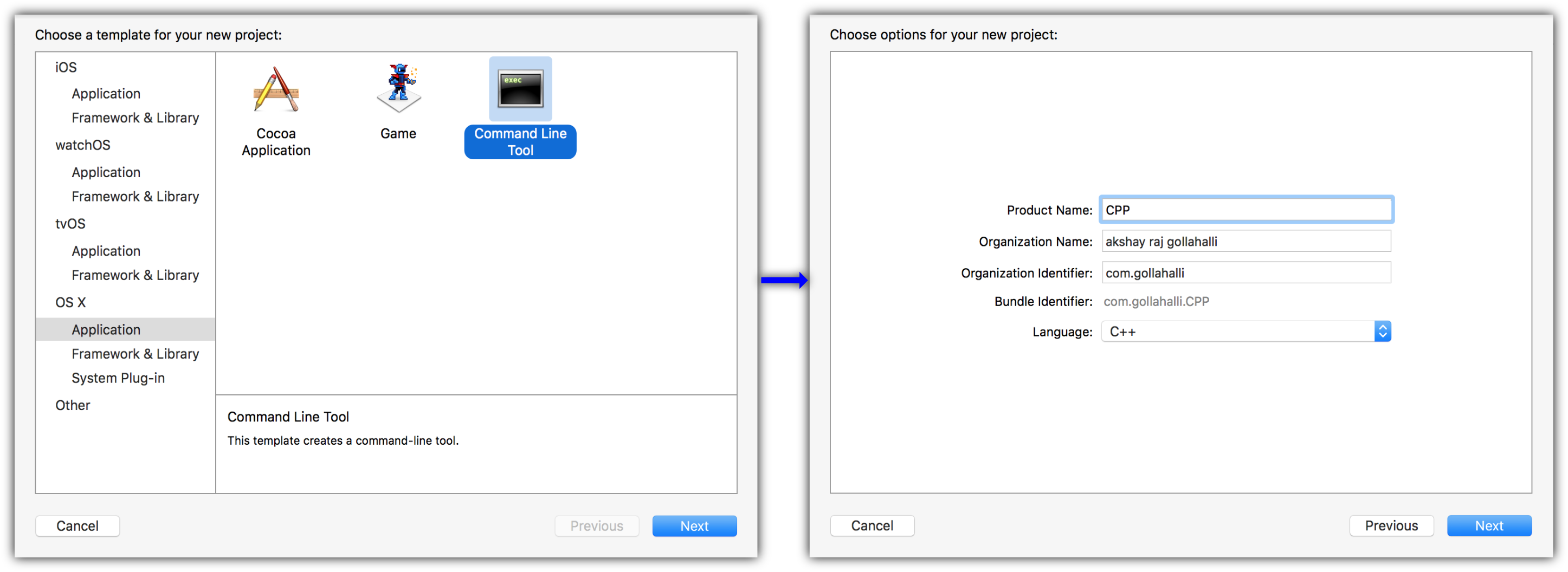 |
| 24 | +3. Save it where every you want. |
| 25 | + |
| 26 | +### 1.2 Windows |
| 27 | + |
| 28 | +1. Go to [https://www.visualstudio.com/en-us/visual-studio-homepage-vs.aspx](https://www.visualstudio.com/en-us/visual-studio-homepage-vs.aspx) and download `Visual Studio Community` version. Make sure `Visual C++` package is selected and continue the installation. |
| 29 | +2. Once installed, Click on `File > New > Project` or use the shortcut `Ctrl + Shift + N`. |
| 30 | + 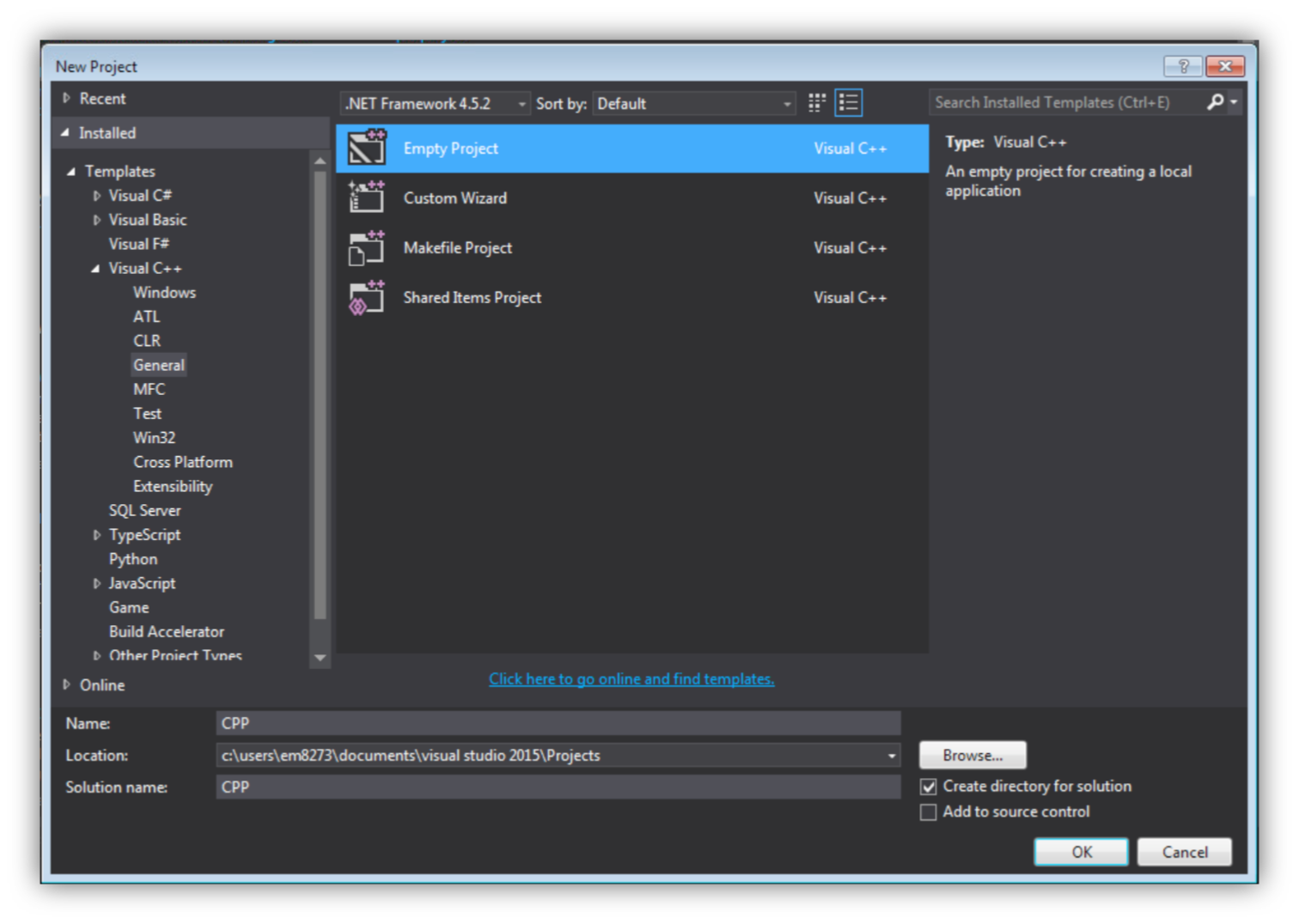 |
| 31 | +3. We are adding the preprocessing variable because of the way Microsoft has written its c++ compiler. For that to happen, we are creating a C++ file. Under `Solution Explorer` right click on `Source Files > Add > New Item...` name it as `test.cpp` and type int he following: |
| 32 | + ```cpp |
| 33 | + #include <cstdio> |
| 34 | + |
| 35 | + using namespace std; |
| 36 | + |
| 37 | + int main(int argc, char ** argv) { |
| 38 | + puts("hello"); |
| 39 | + return 0; |
| 40 | + } |
| 41 | + ``` |
| 42 | + From the menubar click on `Build > Build Solution` |
| 43 | +4. Under Solution Explorer, right click on `CPP > Properties` |
| 44 | + 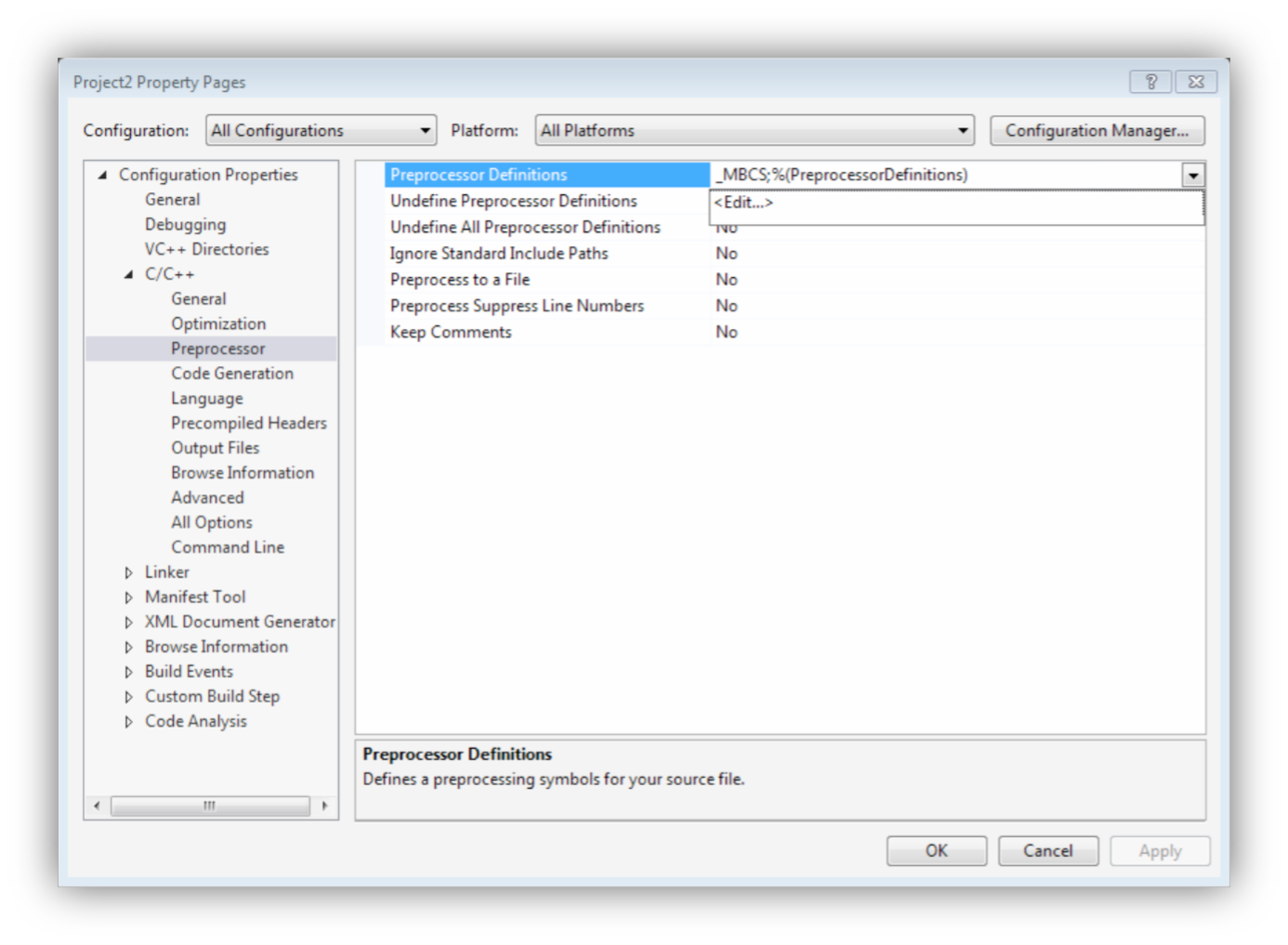 |
| 45 | + As shown in the image above click on `<Edit...>`. In the text box type in the following: |
| 46 | + ``` |
| 47 | + _CRT_SECURE_NO_WARNINGS |
| 48 | + _HAS_ITERATOR_DEBUGGING=0 |
| 49 | + ``` |
| 50 | + Click on `OK` |
| 51 | +
|
| 52 | +**Running the CPP files** |
| 53 | +
|
| 54 | +1. Open your command prompt, by doing `WINDOWS + R` and type in `cmd`. |
| 55 | +2. To run the built `test.cpp`, you would have to go to the project folder `CPP > Build > CPP.exe`. Drag and drop `CPP.exe` on the command prompt the press `Enter`, this will output `hello`. |
| 56 | +
|
| 57 | +## 2 Basics |
| 58 | +
|
| 59 | +C++ inherits most of its code style from C language, but both are very different from each other. Lets consider an example: |
| 60 | +
|
| 61 | +```cpp |
| 62 | +// This is a comment |
| 63 | +
|
| 64 | +/* |
| 65 | + This is a block code. |
| 66 | +*/ |
| 67 | +#include <cstdio> //<- Libraries |
| 68 | +#include <iostream> |
| 69 | +
|
| 70 | +using namespace std; //<- scope to identifiers |
| 71 | +
|
| 72 | +int main(int argc, char ** argv) { |
| 73 | + puts("hello"); // this statement outputs hello with a new line |
| 74 | + printf("hello\n"); // this is similar to puts but doesn't end with new line |
| 75 | + cout << "hello\n"; // more complex way to output without new line |
| 76 | + return 0; // 0 means success |
| 77 | +} |
| 78 | +``` |
| 79 | + |
| 80 | +A C++ program can also be written like this (thought I wouldn't recommend it): |
| 81 | + |
| 82 | +```cpp |
| 83 | +#include <cstdio> |
| 84 | +using namespace std; |
| 85 | + |
| 86 | +int |
| 87 | +main |
| 88 | +( |
| 89 | +int |
| 90 | +argc, |
| 91 | +char |
| 92 | +** |
| 93 | +argv) { |
| 94 | +puts(" |
| 95 | +hello") |
| 96 | + ; |
| 97 | + return 0; |
| 98 | +} |
| 99 | +``` |
| 100 | + |
| 101 | +**Things to remember** |
| 102 | + |
| 103 | +1. A statement should always end with `;`. |
| 104 | +2. `#Include` should always be in single line with out any space followed by `<>` or `""`. |
| 105 | + |
| 106 | +### 2.1 Identifiers |
| 107 | + |
| 108 | +C++ follows standard [ISO Latin Alphabets](https://en.wikipedia.org/wiki/ISO_basic_Latin_alphabet), [Western arabic numbers](https://en.wikipedia.org/wiki/Arabic_numerals) and [ASCII Underscore](http://www.theasciicode.com.ar/ascii-printable-characters/underscore-understrike-underbar-low-line-ascii-code-95.html). These identifiers cannot conflict with C++ 86 keywords (which includes 11 tokens) |
| 109 | + |
| 110 | +| alignas (since C++11) | explicit | signed | |
| 111 | +|-------------------------|------------------------|-----------------------------| |
| 112 | +| alignof (since C++11) | export(1) | sizeof | |
| 113 | +| and | extern | static | |
| 114 | +| and_eq | FALSE | static_assert (since C++11) | |
| 115 | +| asm | float | static_cast | |
| 116 | +| auto(1) | for | struct | |
| 117 | +| bitand | friend | switch | |
| 118 | +| bitor | goto | template | |
| 119 | +| bool | if | this | |
| 120 | +| break | inline | thread_local (since C++11) | |
| 121 | +| case | int | throw | |
| 122 | +| catch | long | TRUE | |
| 123 | +| char | mutable | try | |
| 124 | +| char16_t (since C++11) | namespace | typedef | |
| 125 | +| char32_t (since C++11) | new | typeid | |
| 126 | +| class | noexcept (since C++11) | typename | |
| 127 | +| compl | not | union | |
| 128 | +| concept (concepts TS) | not_eq | unsigned | |
| 129 | +| const | nullptr (since C++11) | using(1) | |
| 130 | +| constexpr (since C++11) | operator | virtual | |
| 131 | +| const_cast | or | void | |
| 132 | +| continue | or_eq | volatile | |
| 133 | +| decltype (since C++11) | private | wchar_t | |
| 134 | +| default(1) | protected | while | |
| 135 | +| delete(1) | public | xor | |
| 136 | +| do | register | xor_eq | |
| 137 | +| double | reinterpret_cast | | |
| 138 | +| dynamic_cast | requires (concepts TS) | | |
| 139 | +| else | return | | |
| 140 | +| enum | short | | |
0 commit comments