Commit f1b4f9a
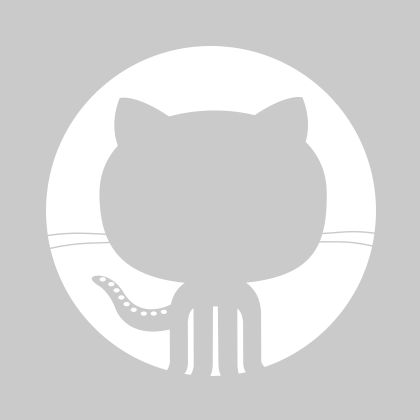
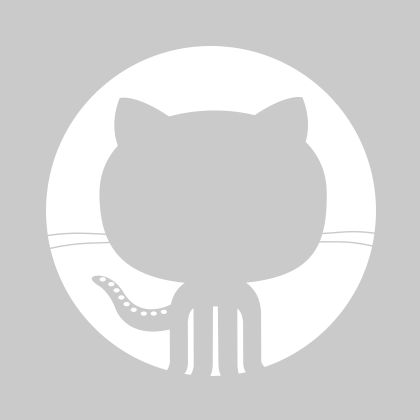
Lars Kappert
Lars Kappert
1 parent 8de6643 commit f1b4f9a
File tree
77 files changed
+3875
-3800
lines changed- code-reuse-patterns
- design-patterns
- dom-and-browser
- function-patterns
- general-patterns
- jquery-patterns
- literals-and-constructors
- object-creation-patterns
Some content is hidden
Large Commits have some content hidden by default. Use the searchbox below for content that may be hidden.
77 files changed
+3875
-3800
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
8 |
| - | |
9 |
| - | |
10 |
| - | |
11 |
| - | |
12 |
| - | |
13 |
| - | |
14 |
| - | |
15 |
| - | |
16 |
| - | |
17 |
| - | |
18 |
| - | |
19 |
| - | |
20 |
| - | |
21 |
| - | |
22 |
| - | |
23 |
| - | |
24 |
| - | |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 |
| - | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
82 | 82 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
8 |
| - | |
9 |
| - | |
10 |
| - | |
11 |
| - | |
12 |
| - | |
13 |
| - | |
14 |
| - | |
15 |
| - | |
16 |
| - | |
17 |
| - | |
18 |
| - | |
19 |
| - | |
20 |
| - | |
21 |
| - | |
22 |
| - | |
23 |
| - | |
24 |
| - | |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
49 | 50 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
8 |
| - | |
9 |
| - | |
10 |
| - | |
11 |
| - | |
12 |
| - | |
13 |
| - | |
14 |
| - | |
15 |
| - | |
16 |
| - | |
17 |
| - | |
18 |
| - | |
19 |
| - | |
20 |
| - | |
21 |
| - | |
22 |
| - | |
23 |
| - | |
24 |
| - | |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
60 | 60 |
|
0 commit comments