Commit c7a476a
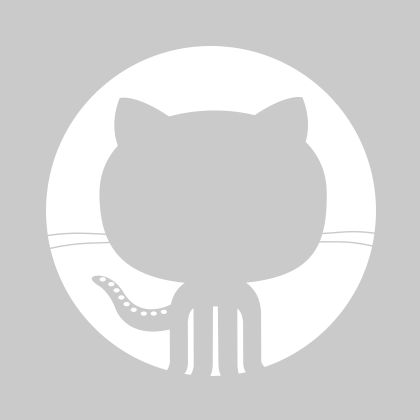
Sommer Matthias (IFAG IT DSA RD CM / External)
1 parent 3d25f61 commit c7a476a
File tree
5 files changed
+43
-13
lines changed- api
- pizza
5 files changed
+43
-13
lines changed+7-4
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
| 8 | + | |
9 | 9 |
| |
10 | 10 |
| |
11 |
| - | |
| 11 | + | |
12 | 12 |
| |
13 | 13 |
| |
14 |
| - | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
15 | 18 |
| |
16 | 19 |
| |
17 | 20 |
| |
18 | 21 |
| |
19 | 22 |
| |
20 |
| - | |
| 23 | + | |
21 | 24 |
| |
22 | 25 |
| |
23 | 26 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
| 4 | + | |
4 | 5 |
| |
5 | 6 |
| |
6 | 7 |
| |
| 8 | + | |
7 | 9 |
| |
8 | 10 |
| |
9 | 11 |
| |
| |||
17 | 19 |
| |
18 | 20 |
| |
19 | 21 |
| |
| 22 | + | |
| 23 | + | |
20 | 24 |
| |
21 | 25 |
| |
22 | 26 |
| |
| |||
73 | 77 |
| |
74 | 78 |
| |
75 | 79 |
| |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + |
+11-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
| |
| 6 | + | |
| 7 | + | |
6 | 8 |
| |
| 9 | + | |
7 | 10 |
| |
8 | 11 |
| |
9 | 12 |
| |
| |||
21 | 24 |
| |
22 | 25 |
| |
23 | 26 |
| |
24 |
| - | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
25 | 30 |
| |
26 |
| - | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
27 | 36 |
|
+10-5
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
15 | 20 |
| |
16 | 21 |
| |
17 | 22 |
| |
| |||
50 | 55 |
| |
51 | 56 |
| |
52 | 57 |
| |
53 |
| - | |
| 58 | + | |
54 | 59 |
| |
55 | 60 |
| |
56 | 61 |
| |
| |||
101 | 106 |
| |
102 | 107 |
| |
103 | 108 |
| |
104 |
| - | |
| 109 | + | |
105 | 110 |
| |
106 | 111 |
| |
107 | 112 |
| |
| |||
129 | 134 |
| |
130 | 135 |
| |
131 | 136 |
| |
132 |
| - | |
| 137 | + | |
133 | 138 |
| |
134 | 139 |
| |
135 | 140 |
| |
| |||
160 | 165 |
| |
161 | 166 |
| |
162 | 167 |
| |
163 |
| - | |
| 168 | + | |
164 | 169 |
| |
165 | 170 |
| |
166 | 171 |
| |
| |||
173 | 178 |
| |
174 | 179 |
| |
175 | 180 |
| |
176 |
| - | |
| 181 | + | |
177 | 182 |
| |
178 | 183 |
| |
179 | 184 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
10 |
| - | |
11 |
| - | |
| 10 | + | |
| 11 | + | |
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
|
0 commit comments