|
1 |
| -FixedMathSharp |
| 1 | +FixedMathSharp-Unity |
2 | 2 | ==============
|
3 | 3 |
|
4 | 4 | 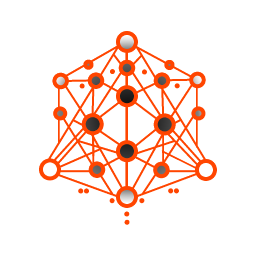
|
5 | 5 |
|
6 |
| -**A high-precision, deterministic fixed-point math library for .NET.** |
7 |
| -Ideal for simulations, games, and physics engines requiring reliable arithmetic without floating-point inaccuracies. |
| 6 | +A **deterministic fixed-point math** library for **Unity** and **.NET**, designed for **lockstep simulation**, **multiplayer determinism**, and **floating-point-free precision**. |
8 | 7 |
|
9 | 8 | ---
|
10 | 9 |
|
11 | 10 | ## 🛠️ Key Features
|
12 | 11 |
|
13 |
| -- **Deterministic Calculations:** Perfect for simulations, multiplayer games, and physics engines. |
14 |
| -- **High Precision Arithmetic:** Uses fixed-point math to eliminate floating-point inaccuracies. |
15 |
| -- **Comprehensive Vector Support:** Includes 2D and 3D vector operations (`Vector2d`, `Vector3d`). |
16 |
| -- **Quaternion Rotations:** Leverage `FixedQuaternion` for smooth rotations without gimbal lock. |
17 |
| -- **Matrix Operations:** Supports transformations with `Fixed4x4` and `Fixed3x3` matrices. |
18 |
| -- **Bounding Shapes:** Includes `IBound` structs `BoundingBox`, `BoundingSphere`, and `BoundingArea` for lightweight spatial calculations. |
19 |
| -- **Advanced Math Functions:** Includes trigonometry and common math utilities. |
20 |
| -- **Unity Integration:** Seamless interoperability with Unity using `FixedMathSharp.Editor`. |
21 |
| - |
| 12 | +- **Fully Deterministic** – Eliminates floating-point errors for lockstep simulations. |
| 13 | +- **Fixed-Point Arithmetic** – Implements `Fixed64` with high precision. |
| 14 | +- **Math & Trigonometry** – Optimized `FixedMath` and `FixedTrigonometry` utilities. |
| 15 | +- **Vector & Matrix Support** – Includes `Vector2d`, `Vector3d`, `FixedQuaternion`, and `Fixed4x4`. |
| 16 | +- **Bounding Volume Utilities** – Use `BoundingBox`, `BoundingSphere`, and `BoundingArea` for collision checks. |
| 17 | +- **Unity Integration** – Compatible with **Unity’s Job System** & **Burst Compiler**. |
22 | 18 |
|
23 | 19 | ---
|
24 | 20 |
|
25 | 21 | ## 🚀 Installation
|
26 | 22 |
|
| 23 | +### **Via Unity Package Manager (UPM)** |
| 24 | +1. Open **Unity Package Manager** (`Window > Package Manager`). |
| 25 | +2. Click **Add package from git URL...**. |
| 26 | +3. Enter: |
27 | 27 |
|
28 |
| -Clone the repository and add it to your project: |
29 |
| - |
30 |
| -### Non-Unity Projects |
31 |
| - |
32 |
| -1. **Install via NuGet**: |
33 |
| - - Add FixedMathSharp to your project using the following command: |
34 |
| - |
35 |
| - ```bash |
36 |
| - dotnet add package FixedMathSharp |
37 |
| - ``` |
38 |
| - |
39 |
| -2. **Or Download/Clone**: |
40 |
| - - Clone the repository or download the source code. |
41 |
| - |
42 |
| - ```bash |
43 |
| - git clone https://github.com/mrdav30/FixedMathSharp.git |
44 |
| - ``` |
45 |
| - |
46 |
| -3. **Add to Project**: |
47 |
| - |
48 |
| - - Include the FixedMathSharp project or its DLLs in your build process. |
49 |
| - |
50 |
| -### Unity |
| 28 | +https://github.com/mrdav30/FixedMathSharp-Unity.git |
51 | 29 |
|
52 |
| -To integrate **FixedMathSharp** into your Unity project: |
53 |
| - |
54 |
| -1. **Download the Package**: |
55 |
| - - Obtain the latest `FixedMathSharp{{VERSION}}.unitypackage` from the [Releases](https://github.com/mrdav30/FixedMathSharp/releases) section of the repository. |
56 |
| - |
57 |
| -2. **Import into Unity**: |
58 |
| - - In Unity, navigate to **Assets > Import Package > Custom Package...**. |
59 |
| - - Select the downloaded `FixedMathSharp{{VERSION}}.unitypackage` file. |
60 |
| - |
61 |
| -3. **Verify the Integration**: |
62 |
| - - After importing, confirm that the `FixedMathSharp` namespace is accessible in your scripts. |
| 30 | +### **Manual Import** |
| 31 | +1. Download the latest `FixedMathSharp.unitypackage` from the [Releases](https://github.com/mrdav30/FixedMathSharp-Unity/releases). |
| 32 | +2. In Unity, go to **Assets > Import Package > Custom Package...**. |
| 33 | +3. Select and import `FixedMathSharp.unitypackage`. |
63 | 34 |
|
64 | 35 | ---
|
65 | 36 |
|
@@ -114,54 +85,11 @@ Console.WriteLine(sinValue); // Output: ~0.707
|
114 | 85 |
|
115 | 86 | ---
|
116 | 87 |
|
117 |
| -## 📦 Library Structure |
118 |
| - |
119 |
| -- **`Fixed64` Struct:** Represents fixed-point numbers for precise arithmetic. |
120 |
| -- **`Vector2d` and `Vector3d` Structs:** Handle 2D and 3D vector operations. |
121 |
| -- **`FixedQuaternion` Struct:** Provides rotation handling without gimbal lock, enabling smooth rotations and quaternion-based transformations. |
122 |
| -- **`IBound` Interface:** Standard interface for bounding shapes `BoundingBox`, `BoundingArea`, and `BoundingSphere`, each offering intersection, containment, and projection logic. |
123 |
| -- **`FixedMath` Static Class:** Provides common math functions. |
124 |
| -- **`FixedTrigonometry` Class:** Offers trigonometric functions using fixed-point math. |
125 |
| -- **`Fixed4x4` and `Fixed3x3`:** Support matrix operations for transformations. |
126 |
| -- **`FixedMathSharp.Editor`:** Extensions for seamless integration with Unity, including property drawers and type conversions. |
127 |
| - |
128 |
| -### Fixed64 Struct |
129 |
| - |
130 |
| -**Fixed64** is the core data type representing fixed-point numbers. It |
131 |
| -provides various mathematical operations, including addition, |
132 |
| -subtraction, multiplication, division, and more. The struct guarantees |
133 |
| -deterministic behavior by using integer-based arithmetic with a |
134 |
| -configurable `SHIFT_AMOUNT`. |
135 |
| - |
136 |
| ---- |
137 |
| - |
138 |
| -## ⚡ Performance Considerations |
139 |
| - |
140 |
| -This library leverages **inline methods** and **fixed-point arithmetic** |
141 |
| -to ensure high precision without the pitfalls of floating-point numbers. |
142 |
| -It is optimized for **deterministic behavior**, making it ideal for physics |
143 |
| -engines, multiplayer simulations, and other time-sensitive applications. |
144 |
| - |
145 |
| ---- |
146 |
| - |
147 |
| -## 🧪 Testing and Validation |
148 |
| - |
149 |
| -Unit tests are used extensively to validate the correctness of mathematical |
150 |
| -operations. Special **fuzzy comparisons** are employed where small precision |
151 |
| -discrepancies might occur, mimicking floating-point behavior. |
152 |
| - |
153 |
| -To run the tests: |
154 |
| -```bash |
155 |
| -dotnet test --configuration Release |
156 |
| -``` |
157 |
| - |
158 |
| ---- |
159 |
| - |
160 | 88 | ## 🛠️ Compatibility
|
161 | 89 |
|
162 |
| -- **.NET Framework:** 4.7.1+ |
163 |
| -- **Unity3D:** Fully compatible with Unity using the `FixedMathSharp.Editor` extension. |
164 |
| -- **Platforms:** Windows, Linux, macOS |
| 90 | +- **.NET Framework:** 4.7.2+ |
| 91 | +- **Unity3D Version:** 2022.3+ |
| 92 | +- **Platforms:** Windows, Linux, macOS, WebGL, Mobile |
165 | 93 |
|
166 | 94 | ---
|
167 | 95 |
|
|
0 commit comments