|
1 |
| -# Segregate 0's and 1's in an Array |
2 |
| - |
3 |
| -## Problem Statement: |
4 |
| -You are given an array of 0s and 1s in random order. Segregate 0s on left side and 1s on right side of the array [Basically you have to sort the array]. |
5 |
| - |
6 |
| -## Algorithm: |
7 |
| -1. Here we will be using 2 indices and initilize the first index `left` as `0` and the last index `right` as `array.length-1` |
8 |
| -2. Run a While left < right loop |
9 |
| -3. Keep incrementing index left while there are 0s at it |
10 |
| -4. Keep decrementing index right while there are 1s at it |
11 |
| -5. If left < right then exchange arr[left] and arr[right] |
12 |
| - |
13 |
| -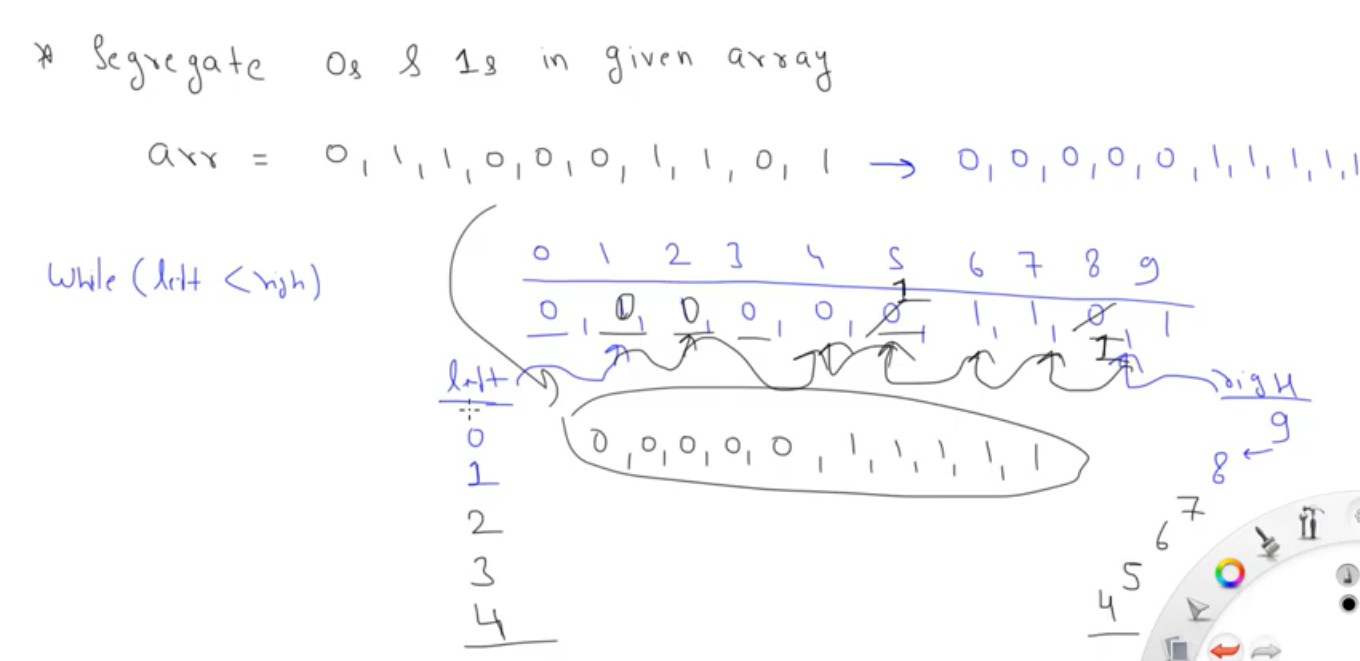 |
14 |
| - |
15 |
| - |
16 |
| -## Code in Java |
17 |
| -```java |
18 |
| - void segregate(int arr[], int size) |
19 |
| - |
20 |
| - { |
21 |
| - /* Initialize left and right*/ as 0 and size-1 |
22 |
| - int left = 0, right = size - 1; |
23 |
| - |
24 |
| - while (left < right) |
25 |
| - { |
26 |
| - /* Increment left index while we see 0 at left */ |
27 |
| - while (arr[left] == 0 && left < right) |
28 |
| - left++; |
29 |
| - |
30 |
| - /* Decrement right index while we see 1 at right */ |
31 |
| - while (arr[right] == 1 && left < right) |
32 |
| - right--; |
33 |
| - |
34 |
| - /* If left is smaller than right then there is a 1 at left |
35 |
| - and a 0 at right. Exchange arr[left] and arr[right]*/ |
36 |
| - if (left < right) |
37 |
| - { |
38 |
| - arr[left] = 0; |
39 |
| - arr[right] = 1; |
40 |
| - left++; |
41 |
| - right--; |
42 |
| - } |
43 |
| - } |
44 |
| - } |
45 |
| - |
46 |
| - /*main*/ |
47 |
| - public static void main(String[] args) |
48 |
| - { |
49 |
| - Segregate obj = new Segregate(); |
50 |
| - int arr[] = new int[]{0, 1, 0, 1, 1, 1}; |
51 |
| - int i, arr_size = arr.length; |
52 |
| - |
53 |
| - obj.segregate(arr, arr_size); |
54 |
| - |
55 |
| - System.out.print("Array after segregation is "); |
56 |
| - for (i = 0; i < 6; i++) |
57 |
| - System.out.print(arr[i] + " "); |
58 |
| - } |
59 |
| -} |
60 |
| -``` |
61 |
| -## Complexity Analysis |
62 |
| - |
63 |
| -Time Complexity: O(n), where n is elements of array |
64 |
| - |
65 |
| -Space Complexity: O(1) |
66 |
| - |
67 |
| -## Miscelleneous |
68 |
| - |
69 |
| -Problem Statement: https://www.geeksforgeeks.org/segregate-0s-and-1s-in-an-array-by-traversing-array-once/ |
70 |
| - |
71 |
| -Reference Video: https://youtu.be/HwDiGWwp11k |
| 1 | +# Segregate 0's and 1's in an Array |
| 2 | + |
| 3 | +## Problem Statement: |
| 4 | +You are given an array of 0s and 1s in random order. Segregate 0s on left side and 1s on right side of the array [Basically you have to sort the array]. |
| 5 | + |
| 6 | +## Algorithm: |
| 7 | +1. Here we will be using 2 indices and initilize the first index `left` as `0` and the last index `right` as `array.length-1` |
| 8 | +2. Run a While left < right loop |
| 9 | +3. Keep incrementing index left while there are 0s at it |
| 10 | +4. Keep decrementing index right while there are 1s at it |
| 11 | +5. If left < right then exchange arr[left] and arr[right] |
| 12 | + |
| 13 | +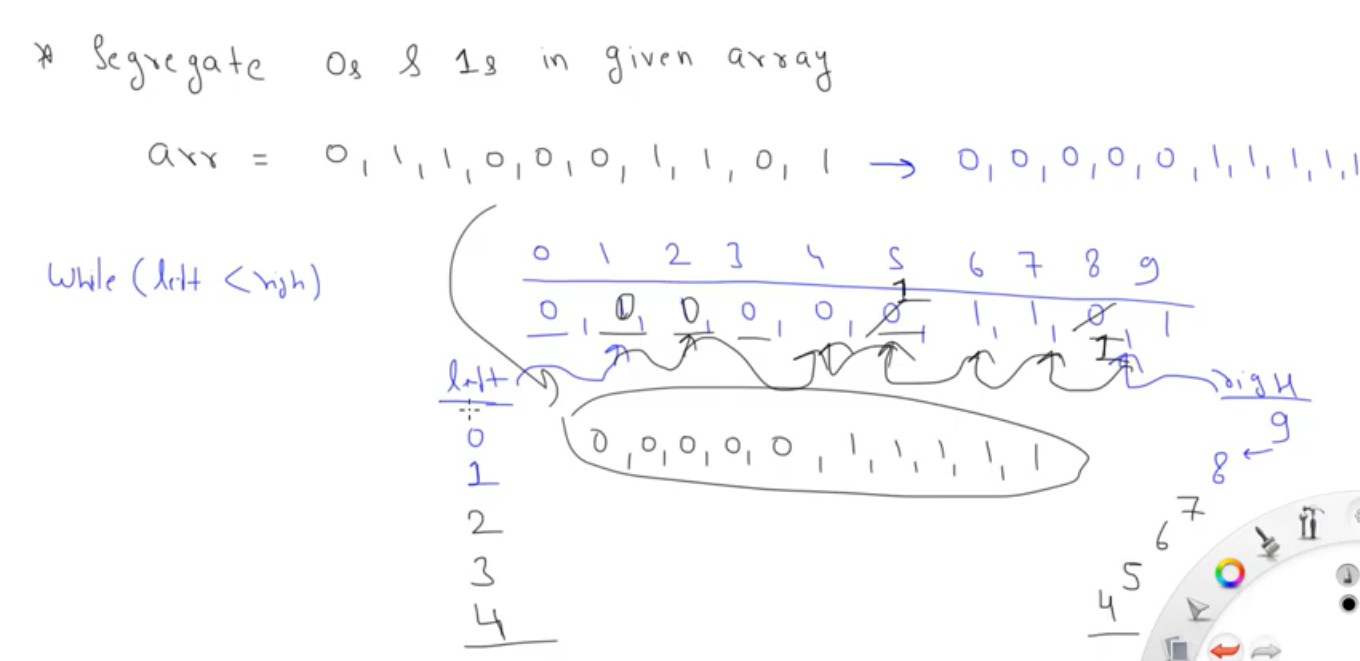 |
| 14 | + |
| 15 | + |
| 16 | +## Code in Java |
| 17 | +```java |
| 18 | + void segregate(int arr[], int size) |
| 19 | + |
| 20 | + { |
| 21 | + /* Initialize left and right*/ as 0 and size-1 |
| 22 | + int left = 0, right = size - 1; |
| 23 | + |
| 24 | + while (left < right) |
| 25 | + { |
| 26 | + /* Increment left index while we see 0 at left */ |
| 27 | + while (arr[left] == 0 && left < right) |
| 28 | + left++; |
| 29 | + |
| 30 | + /* Decrement right index while we see 1 at right */ |
| 31 | + while (arr[right] == 1 && left < right) |
| 32 | + right--; |
| 33 | + |
| 34 | + /* If left is smaller than right then there is a 1 at left |
| 35 | + and a 0 at right. Exchange arr[left] and arr[right]*/ |
| 36 | + if (left < right) |
| 37 | + { |
| 38 | + arr[left] = 0; |
| 39 | + arr[right] = 1; |
| 40 | + left++; |
| 41 | + right--; |
| 42 | + } |
| 43 | + } |
| 44 | + } |
| 45 | + |
| 46 | + /*main*/ |
| 47 | + public static void main(String[] args) |
| 48 | + { |
| 49 | + Segregate obj = new Segregate(); |
| 50 | + int arr[] = new int[]{0, 1, 0, 1, 1, 1}; |
| 51 | + int i, arr_size = arr.length; |
| 52 | + |
| 53 | + obj.segregate(arr, arr_size); |
| 54 | + |
| 55 | + System.out.print("Array after segregation is "); |
| 56 | + for (i = 0; i < 6; i++) |
| 57 | + System.out.print(arr[i] + " "); |
| 58 | + } |
| 59 | +} |
| 60 | +``` |
| 61 | +## Complexity Analysis |
| 62 | + |
| 63 | +Time Complexity: O(n), where n is elements of array |
| 64 | + |
| 65 | +Space Complexity: O(1) |
| 66 | + |
| 67 | +## Miscelleneous |
| 68 | + |
| 69 | +Problem Statement: https://www.geeksforgeeks.org/segregate-0s-and-1s-in-an-array-by-traversing-array-once/ |
| 70 | + |
| 71 | +Reference Video: https://youtu.be/HwDiGWwp11k |
0 commit comments