-
Notifications
You must be signed in to change notification settings - Fork 117
Controls
Controls in Comet are lightweight. In order to keep them lightweight, each control has only 1-2 read-only constructor parameters.
View
is the base type for all UI in Comet. Views are composable and can have a Body
. The body can be specified by the [Body]
attribute, or by setting the Body
function.
Attribute:
class MyView : View
{
[Body]
View view() => new VStack{
new Text("Hello World")
};
}
Function:
class MyView : View
{
public MyView()
{
Body = () => new VStack{
new Text("Hello World")
};
}
}

Text is used to display a string on the screen.
Constructor Parameters
Name | Type | Description |
---|---|---|
Value | String | The text value to be displayed |
Example:
new Text("Hello World")

A button is used to display a string on the screen.
Constructor Parameters
Name | Type | Description |
---|---|---|
Text | String | The text value to be displayed |
Clicked | Action | The action that fires when clicked/tapped |
Example:
new Button("Click me!",()=> Console.WriteLine("I was clicked"))

Displays a loading indicator.
Constructor Parameters
Name | Type | Description |
---|
Example:
new ActivityIndicator()

Constructor Parameters
Name | Type | Description |
---|---|---|
IsChecked | bool | Toggles the checked state |
Example:
new CheckBox(true)
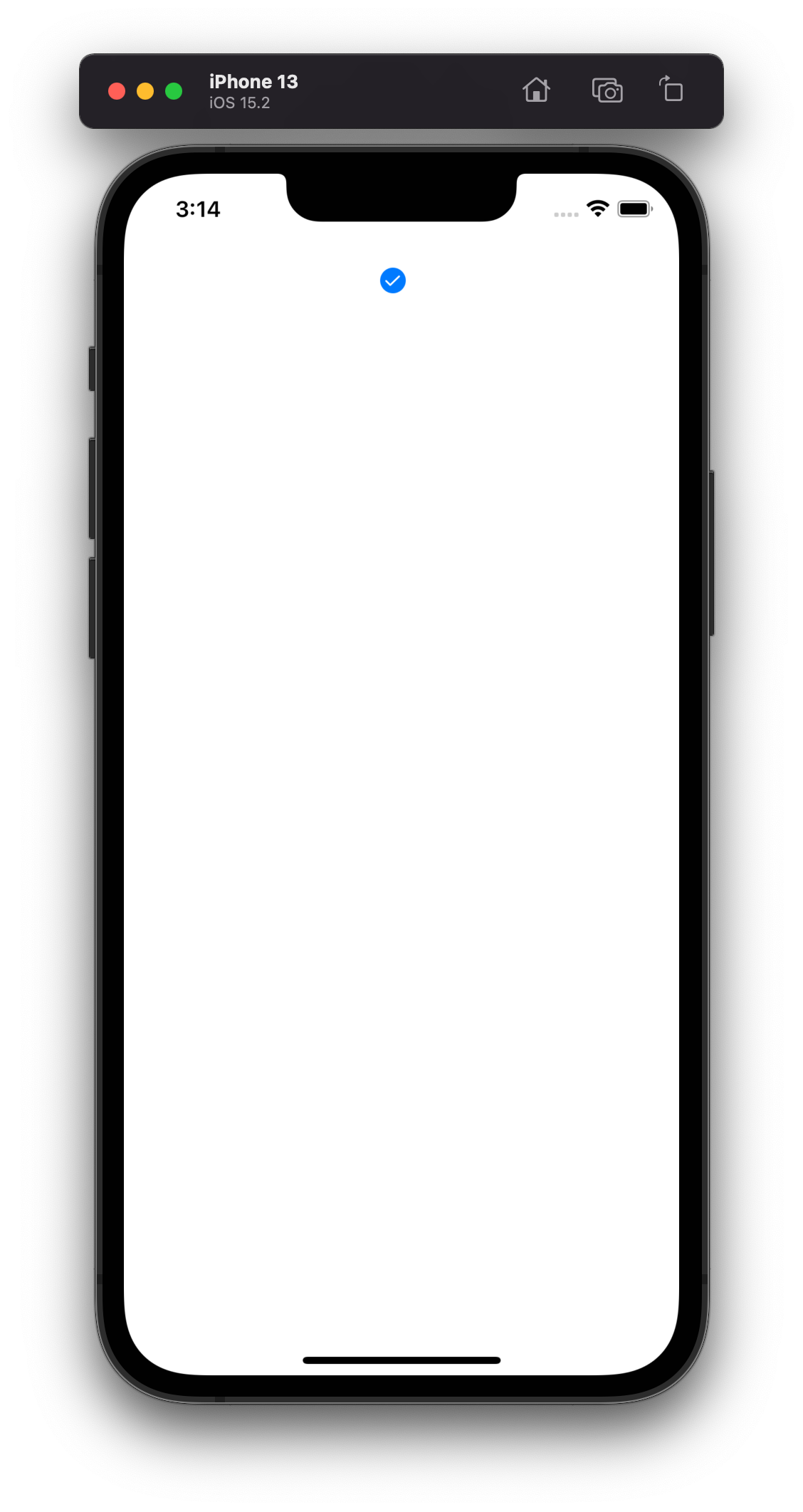
A Datepicker.
Constructor Parameters
Name | Type | Description |
---|---|---|
Date | DateTime | The current value |
MinimumDate | DateTime | The Minimum value |
MaximumDate | DateTime | The Maximum value |
Example:
new DatePicker(DateTime.Today)

Displays an image.
Constructor Parameters
Name | Type | Description |
---|---|---|
ImageSource | String,ImageSource | This value can be a string or an ImageSource. If its a string it can be a file path or url |
Example:
new Image("comet_logo.png")

Displays a scrollable Vertical list of items. ListView can be virtualized, or passed in an existing ICollection.
Constructor Parameters
Name | Type | Description |
---|---|---|
Items | ICollection | Optional, can be virtualized instead. If this is an ObservableCollection, the List will auto update. |
Example:
Virtualized
new ListView<int>
{
Count = () => 100,
ItemFor = (i) => i,
ViewFor = (i) => new Text($"{i}")
};
Using List
new ListView<int>(Enumerable.Range(0,100).ToList())
{
ViewFor = (i) => new Text($"{i}")
};
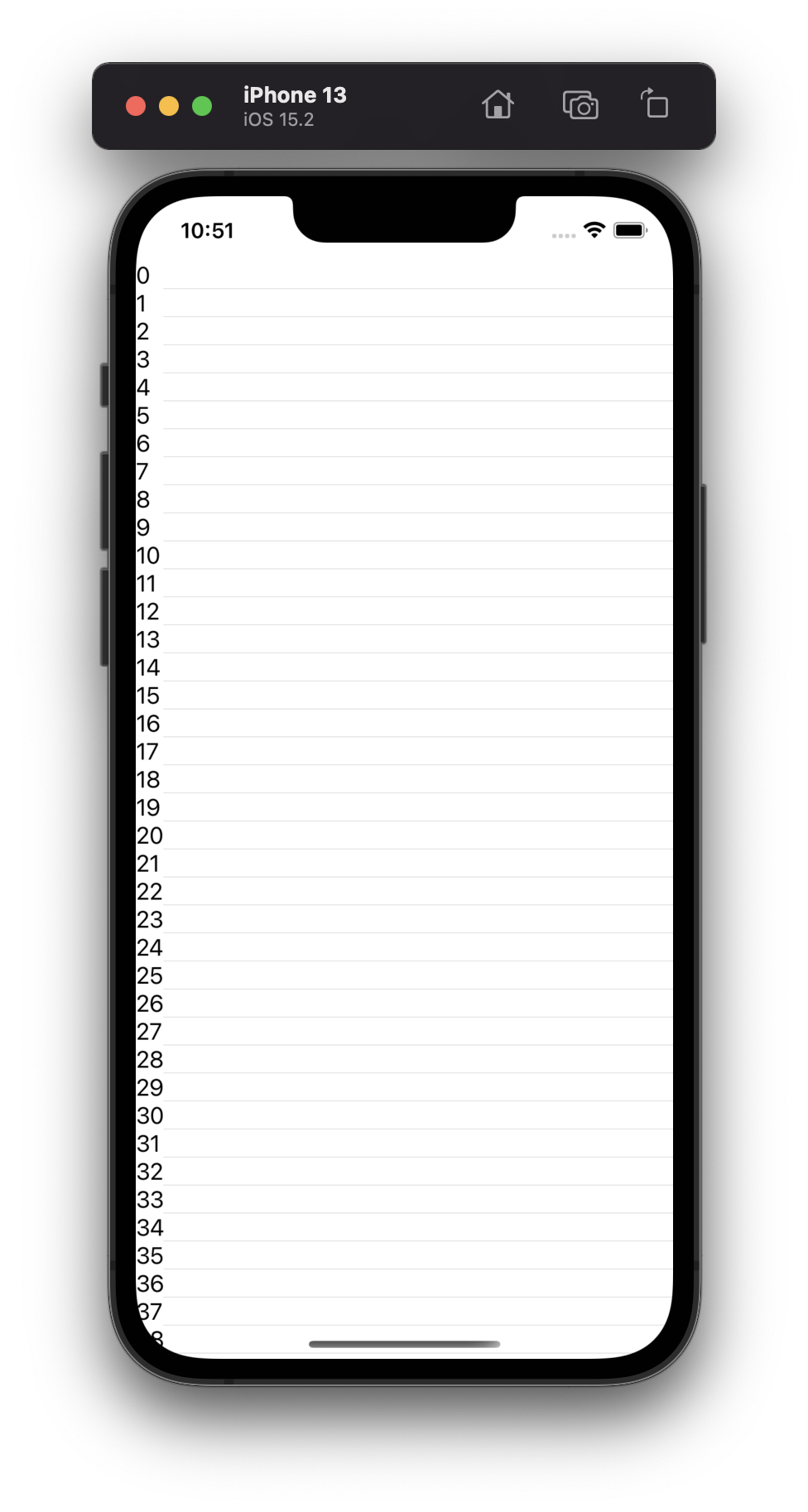