-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: add "reverse-linked-list" content
- Loading branch information
Showing
3 changed files
with
136 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,135 @@ | ||
--- | ||
layout: doc | ||
--- | ||
|
||
# 206-反转链表 | ||
|
||
标签:<Badge type="tip" text="递归" /> <Badge type="tip" text="链表" /> | ||
|
||
## 题目信息 | ||
|
||
**题目地址**: [206. 反转链表](https://leetcode.cn/problems/reverse-linked-list/description/) | ||
|
||
**题目内容:** | ||
|
||
```javascript | ||
给你单链表的头节点head,请你反转链表,并返回反转后的链表。 | ||
``` | ||
> 示例 1: | ||
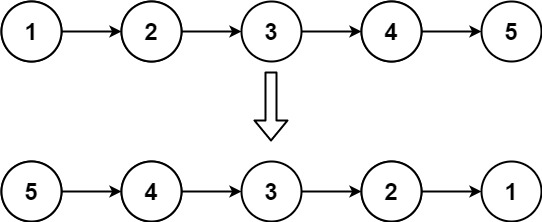 | ||
|
||
> 输入:head = [1,2,3,4,5] | ||
> | ||
> 输出:[5,4,3,2,1] | ||
> 示例 2: | ||
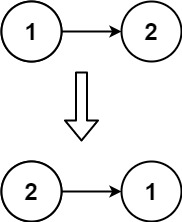 | ||
|
||
> 输入:head = [1,2] | ||
> | ||
> 输出:[2,1] | ||
> 示例 3: | ||
> 输入:head = [] | ||
> | ||
> 输出:[] | ||
```javascript | ||
提示: | ||
|
||
链表中节点的数目范围是 [0, 5000] | ||
-5000 <= Node.val <= 5000 | ||
|
||
进阶:链表可以选用迭代或递归方式完成反转。你能否用两种方法解决这道题? | ||
``` | ||
|
||
## 题解 | ||
|
||
### 解法一 | ||
|
||
**思路:** | ||
|
||
> 使用`双指针`思路,定义`prev`指针默认为`null`,`cur`指针默认指向`head`,遍历链表,然后确定`cur`和`prev`直接的指向关系,然后`向右移动两个指针`,直到`cur === null`的时候结束 | ||
> | ||
> `temp`的存在是因为我们将`cur.next`指向`prev`后,向右移动`cur`时,会找不到需要移动的位置,所以需要现将`cur在原始链表中的next`暂时记录下来,每次循环`temp`的值都会改变 | ||
> | ||
> 赋值的顺序为: | ||
> | ||
> 1、先声明`temp = cur.next` | ||
> | ||
> 2、将`cur.next`指向`prev` | ||
> | ||
> 3、`prev`赋值为`cur`(`prev = cur)` | ||
> | ||
> 4、将`cur`赋值为`temp` | ||
> | ||
> 最终的反转结果就是`绿色箭头`所展现的,图示如下: | ||
|
||
 | ||
|
||
**代码实现:** | ||
|
||
```javascript | ||
/** | ||
* Definition for singly-linked list. | ||
* function ListNode(val, next) { | ||
* this.val = (val===undefined ? 0 : val) | ||
* this.next = (next===undefined ? null : next) | ||
* } | ||
*/ | ||
/** | ||
* @param {ListNode} head | ||
* @return {ListNode} | ||
*/ | ||
var reverseList = function(head) { | ||
let curNode = head | ||
let prevNode = null | ||
while(curNode !== null) { | ||
const tempNode = curNode.next | ||
curNode.next = prevNode | ||
prevNode = curNode | ||
curNode = tempNode | ||
} | ||
return prevNode | ||
}; | ||
``` | ||
|
||
### 解法二 | ||
|
||
**思路:** | ||
|
||
> 使用`递归`写法,可以参照上面的`双指针`写法来,就很容易理解 | ||
**代码实现:** | ||
|
||
```javascript | ||
/** | ||
* Definition for singly-linked list. | ||
* function ListNode(val, next) { | ||
* this.val = (val===undefined ? 0 : val) | ||
* this.next = (next===undefined ? null : next) | ||
* } | ||
*/ | ||
/** | ||
* @param {ListNode} head | ||
* @return {ListNode} | ||
*/ | ||
var reverseList = function(head) { | ||
const reverseLinklist = (prev, cur) => { | ||
if(cur === null) return prev | ||
const temp = cur.next | ||
cur.next = prev | ||
/** | ||
下面reverseLinklist函数的传参就相当于方法一中的这两行代码 | ||
prevNode = curNode | ||
curNode = tempNode | ||
*/ | ||
return reverseLinklist(cur, temp) | ||
} | ||
return reverseLinklist(null, head) | ||
}; | ||
``` |
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.